Here I’ll be posting small scripts that are not big enough to deserve their own page. These scripts can be run using TamperMonkey
Persistent build menu
Approved for tribalwars.nl under t13903626
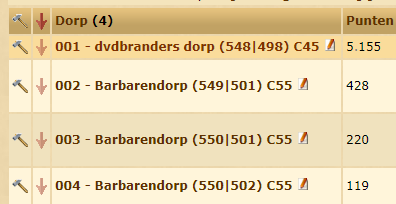
In the premium account overview, there’s a building menu. Clicking on the hammer, followed by clicking on the level of a building, lets you upgrade that building without having to go into the main building of the given village. The only annoying thing, you have to click the hammer every time you enter this page. This script solves that, by saving the state of the hammer.
UserScript
// ==UserScript==
// @name Show all upgrades persisent
// @namespace https://daniel.dmvandenberg.nl/scripting-tribal-wars
// @version 0.1
// @description try to take over the world!
// @author Daniël van den Berg
// @include https://*.tribalwars.*/game.php?village=*&screen=overview_villages&mode=buildings*
// @grant none
// ==/UserScript==
(function() {
'use strict';
var BuildingOverview = window.BuildingOverview;
var sUniqueLocalStorageKey = "BuildingOverview.show_all_upgrade_buildings.262f1ae4-484e-4021-9f24-f39be850c5d5";
BuildingOverview.show_all_upgrade_buildings_base = BuildingOverview.show_all_upgrade_buildings;
BuildingOverview.show_all_upgrade_buildings = ()=>{
BuildingOverview.show_all_upgrade_buildings_base();
window.localStorage[sUniqueLocalStorageKey] = BuildingOverview._display_all;
}
if (window.localStorage[sUniqueLocalStorageKey] == "true"){
BuildingOverview.show_all_upgrade_buildings()
}
})();
Scavenge templates
Approved for tribalwars.nl under T13907137, hotkeys approved under T13916389.
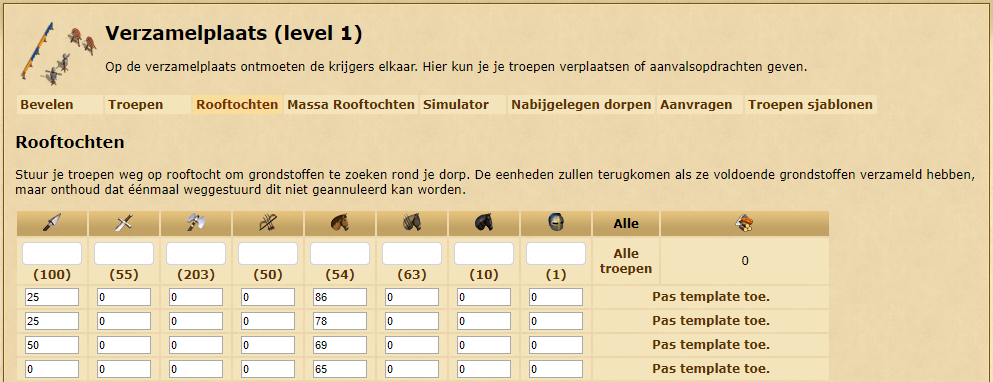
Provides a means of saving templates for scavenge hunts, saved per village. This script also provides 5 hotkeys: u,i,o,p to fill in the first, second, third and fourth template respectively, and y to send out the right-most non-active scavenge hunt. (This means that the key combination “pyoyiyuy” sends out all scavenges with their respective templates.)
UserScript
// ==UserScript==
// @name Scavenge template
// @namespace https://daniel.dmvandenberg.nl/scripting-tribal-wars
// @version 0.2
// @description try to take over the world!
// @author Daniël van den Berg
// @match https://*.tribalwars.nl/game.php*screen=place*
// @grant none
// ==/UserScript==
(function() {
'use strict';
let sUniquePrefix = "95c9ffba-8927-4f77-9af0-269d33e1d770_";
let game_data = window.game_data;
function getVillage(iVillage){
if (!iVillage){iVillage = game_data.village.id;}
if (!window.localStorage[sUniquePrefix + iVillage]){
window.localStorage[sUniquePrefix + iVillage] = "{}";
}
try{
return JSON.parse(window.localStorage[sUniquePrefix + iVillage] || "{}");
} catch (e){ return {} }
}
function getStorage(sStorage, iVillageID){
return getVillage(iVillageID)[sStorage] || {};
}
function setStorage(sStorage, oObject){
let iVillage = game_data.village.id;
let oNew = getVillage();
oNew[sStorage] = oObject;
window.localStorage[sUniquePrefix + iVillage] = JSON.stringify(oNew);
}
let tTableSquad = document.querySelector("#scavenge_screen table.candidate-squad-widget tbody");
if (!tTableSquad){
return;
}
let oScavengeSquad = getStorage("oScavengeSquad");
for (let iRaid = 0; iRaid < 4; iRaid++) {
let trRow = document.createElement("tr");
trRow.id = `scavenge-${iRaid}`;
tTableSquad.appendChild(trRow);
let oSquad = oScavengeSquad[iRaid] || {};
for (let iUnit = 0; iUnit < tTableSquad.querySelectorAll(".unit_link").length; iUnit++) {
let tdNumber = document.createElement("td");
trRow.appendChild(tdNumber);
let eInput = document.createElement("input");
tdNumber.appendChild(eInput);
eInput.name = `${iUnit}`;
eInput.value = oSquad[iUnit] || 0;
eInput.type = "number";
eInput.id = `scavenge-${iRaid}-${iUnit}`;
eInput.addEventListener("change", (eTarget) => {
oSquad[eTarget.target.name] = eTarget.target.value * 1;
oScavengeSquad[iRaid] = oSquad;
setStorage("oScavengeSquad", oScavengeSquad);
});
}
let tdFill = document.createElement("td");
trRow.appendChild(tdFill);
tdFill.colSpan = 2;
let eFill = document.createElement("a");
tdFill.appendChild(eFill);
eFill.innerText = "Pas template toe.";
let fnClick = ()=>{
for (let iUnit = 0; iUnit < tTableSquad.querySelectorAll(".unit_link").length; iUnit++) {
let iNewValue = trRow.children[iUnit].children[0].value * 1;
let iMaxValue = tTableSquad.children[1].children[iUnit].children[1].innerText.replace("(","").replace(")","") * 1;
if (iNewValue > iMaxValue){
alert(`Niet genoeg troepen`);
return;
}
}
for (let iUnit = 0; iUnit < tTableSquad.querySelectorAll(".unit_link").length; iUnit++) {
let iNewValue = trRow.children[iUnit].children[0].value * 1;
tTableSquad.children[1].children[iUnit].children[0].value = iNewValue;
var evt = document.createEvent("HTMLEvents");
evt.initEvent("change", false, true);
tTableSquad.children[1].children[iUnit].children[0].dispatchEvent(evt);
}
};
document.addEventListener("keydown", (e)=>{
if(e.key == "u" && iRaid == 0) fnClick();
if(e.key == "i" && iRaid == 1) fnClick();
if(e.key == "o" && iRaid == 2) fnClick();
if(e.key == "p" && iRaid == 3) fnClick();
});
eFill.addEventListener("click", fnClick);
}
document.addEventListener("keydown", (e)=>{
if(e.key == "y"){
try{
Array.from(document.querySelectorAll(".free_send_button")).last().click();
}
catch(e){}
}
});
})();